EFENTO Sensors
Describes how to integrate the sensor portfolio of EFENTO with your application.
EFENTO provides a wide range of NB-IoT sensors to measure temperature, humidity, CO2, pressure, gas, water leakage, open/close, pulse counts, etc.. On demand they can be provided as Ready for IoT Creators and be shipped with an IoT Creators SIM card and pre-integrated with IoT Creators SCS.
In this chapter you get explained how you can register EFENTO sensors in the IoT Creators portal and to how you can decode the sensor data to integrate it with your application or IoT platform.
Contact EFENTO directly
In case of any questions which are very specific to EFENTO devices please contact directly sklep (at) efento.pl.
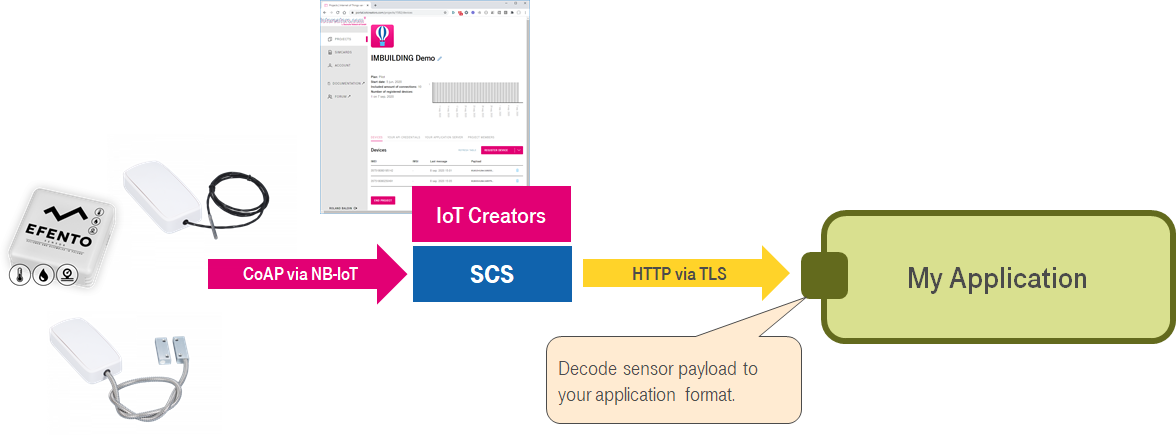
All Efento NB-IoT sensors using CoAP as IoT communication protocoll which is integrated with IoT Creators SCS middleware. The sensors provide the measurement values as a single data package in hex format. This data is forwarded by the SCS to your application URL as the value element as part of the following JSON payload.
{
"reports":[{
"serialNumber":"IMEI:351938100106687",
"timestamp":1600439712734,
"subscriptionId":"fa37d89c-a7e2-4f3d-b12f-6002a3642b4c",
"resourcePath":"uplinkMsg/0/data",
"value":"0a06282c02403cf61001183c22170801108c9cfc860620a2042a0a0100000101000100000022160802108c9cfc860620602a0a000103030100010201002885a8fc8606381a4001482582010f383637393937303331393539343133"
}],
"registrations":[],
"deregistrations":[],
"updates":[],
"expirations":[],
"responses":[]
}
To get the measurement values of the sensor into your application you need to decode the hex data of the value element to the actual measurement values.
The objects which are exchanged with CoAP between the sensor and the application are serialized with the protobuf format from google. That requires:
- the basic protobuf and
- six libraries and
- the EFENTO specific message type definitions.
Before you can integrate IoT Creators with your application and implement the EFENTO message decoding within your application you need to register EFENTO sensor devices in an IoT Creators project.
Since the CoAP protocol integration of IoT Creators SCS is still in a test phase the IoT Creators portal doesn't support the interactive registration of CoAP devices such as the ones of EFENTO. That's why you can only register EFENTO's CoAP devices with the IoT Creators API.
In the following I will explain first how you can register CoAP devices with IoT Creators API and after this how you can implement the message decoding for your application.
How to register EFENTO Sensors with IoT Creators API
To register an EFENTO Sensor as CoAP device with the IoT Creators API you need to use the API credentials which are given in the IoT Creators project in the tab "YOUR API CREDENTIALS".
Use API credentials to register CoAP device.
With those credentials perform the following steps.
1. Register your application URL for the API user
You need to register at least a dummy application URL for the API user because otherwise it will not be possible to create new devices via the API.
REQUEST PUT https://api.scs.iot.telekom.com:443/m2m/applications/registration
REQUEST HEADERS: {
'Content-Type': 'application/json',
'Accept': 'application/json',
'Authorization': 'Basic <base64(API-USER:API-USER-PASSWORD)>'
}
REQUEST BODY: {
'url': 'https://dev.scs.iot.telekom.com/scs-callback-dummy',
'headers': {
'key1':'value1'
}
}
RESPONSE BODY: {
"msg": "Success",
"code": 1000
}
2. Create the Std. CoAP device in IoT Creators
You find the username and the password of your API user in the page "YOUR APPLICATION SERVER" of your IoT Creators Projects. You find there also the "groupName" for the request body in this page as well.
You can add a list of key/value pairs as addtional "customAttributes" to the device. Those key/value pairs are passed along with each message of the device to the configured application URL.
REQUEST POST https://api.scs.iot.telekom.com:443/m2m/endpoints
REQUEST HEADERS: {
'Content-Type': 'application/json',
'Accept': 'application/json',
'Authorization': 'Basic <base64(API-USER:API-USER-PASSWORD)>'
}
REQUEST BODY: {
'serialNumber': 'IMEI:512668894698385',
'groupName': 'CON_0000002299',
'protocol': 'HTTP',
'additionalParams': {
'adaptationLayerName': 'STDCOAP_TEST'
},
'address': '',
'identifier': '',
'customAttributes': {
'deviceType': 'Efento'
}
}
RESPONSE BODY: {
"msg": "Device added successfully",
"code": 3000
}
3. Create subscription for the Std. CoAP device
Because the support for Standard CoAP devices are not 100% finished in the IoT Creators portal I recommend to add the suscription with the API as well.
REQUEST POST https://api.scs.iot.telekom.com:443/m2m/subscriptions?type=resources
REQUEST HEADERS: {
'Content-Type': 'application/json',
'Accept': 'application/json',
'Authorization': 'Basic <base64(API-USER:API-USER-PASSWORD)>'
}
REQUEST BODY: {
'subscriptionType': 'resources',
'groupName': 'CON_0000002299',
'deletionPolicy': 0,
'resources': [
{
'conditions': {},
'resourcePath': 'uplinkMsg/0/data'
},
{
'conditions': {},
'resourcePath': 'downlinkMsg/0/data'
}
],
'criteria': {
'serialNumbers': ['IMEI:512668894698385']
}
}
RESPONSE BODY: {
"subscriptionId": "8974985d-fa8c-472d-8ae3-202e18143e1d",
"msg": "Success",
"code": 1000
}
How to decode EFENTO Sensor Payload with Python
1. Step: Install protobuf and six packages
To install the required protobuf and six packages perform the following commands:
$ pip install protobuf
$ pip install six
2. Step: Download EFENTO specific protobuf message type defintions
Create a working test directory and download all python files "efento_*.py" of the EFENTO specific message type definitions from https://github.com/iotcreators/doclib-downloads/tree/master/device-integrations/efento in it.
3. Step: Create test script to call EFENTO message decoder
In your working directory create the following executable python script:
#!/usr/bin/env python3
import sys
import argparse
import json
import logging
import traceback
import efento_decoder
log = logging.getLogger(__name__)
TESTDATA = "0a06282c02403cf61001183c2217080110f48cf386062096042a0a000000000000000000002216080210f48cf3860620642a0a0000000000000000000028ed98f38606381a4001482582010f383637393937303331393539343133"
if __name__ == '__main__':
log.debug("*** START ***")
cmdlineParser = argparse.ArgumentParser(prog="test_efento_decoder.py",
description="Decodes sensor payload.")
cmdlineParser.add_argument("-loglevel", metavar='<loglevel>',
choices=["INFO", "WARN", "DEBUG"], default="INFO",
help="Log level.Possible values are INFO, WARN, DEBUG.")
cmdlineParser.add_argument("payload", nargs='*',
help="Payload")
ns = cmdlineParser.parse_args()
logging.basicConfig(force=True, level=ns.loglevel, format="%(asctime)s | %(levelname)s | %(name)s | %(message)s")
log.debug("loglevel = %s" % (str(ns.loglevel)))
log.debug("payload = %s" % (str(ns.payload)))
exitCode = 0
try:
if not ns.payload or ns.payload[0] == "TESTDATA":
payload = TESTDATA
else:
payload = ns.payload[0]
d = efento_decoder.decode(payload)
log.info(json.dumps(d, sort_keys=True, indent=4))
except Exception as ex:
log.error(str(ex))
exitCode = 1
traceback.print_exc()
finally:
log.debug("*** END OK ***")
sys.exit(exitCode)
This script imports and executes the main module efento_decoder which contains the main function of the decoder.
usage: test_efento_decoder.py [-h] [-loglevel <loglevel>] [payload [payload ...]]
Decodes sensor payload from EFENTO sensors.
positional arguments:
payload Payload
optional arguments:
-h, --help show this help message and exit
-loglevel <loglevel> Log level.Possible values are INFO, WARN, DEBUG.
As you can see the test script contains a hex message as sample data. By this the script can be executed without any command line arguments:
$ ./test_efento_decoder.py
To test the decoder and to play around with it you can generate sample payload with the EFENTO tool https://dev.efento.io/tools/protocol/. With this tool you can generate payload data for any sensor of EFENTO.
EFENTO Measurement Types
The following measurement types with its value ranges, resolutions, units are supported by the decoder.
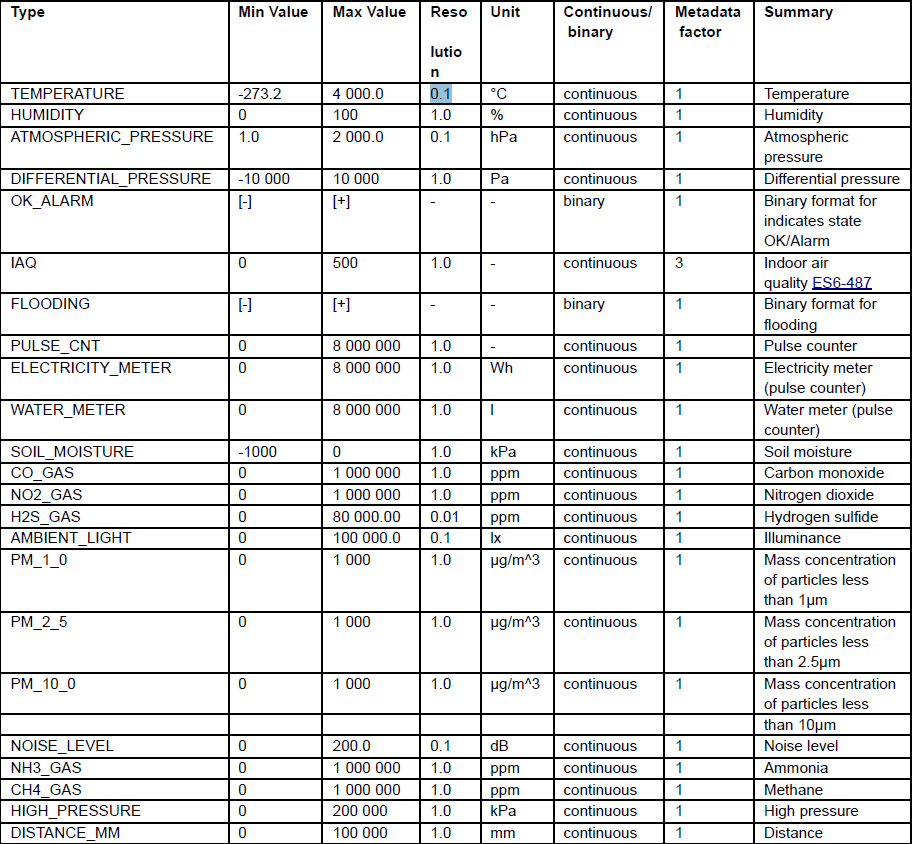
Updated almost 3 years ago