Withthegrid
Learn how to integrate IoT Creators with the Withthegrid platform.
Also available as a video!
In case you prefer audiovisual tutorials, feel free to check out Withthegrid's video for this toturial.
Steps
- Creating environments (monitoring & connectivity)
- Creating a new webhook
- Creating a new device type
- Creating a new report type and quantities
- Configuring IoT Creators webhook
- Configuring device type
- Configuring IoT Creators and registering the device
- Configuring report type
- Claiming device tokens
- Configuring the monitoring environment
Architecture
The Withthegrid asset monitoring platform has two types of environments:
- Monitoring environments: Where the end users obtain an overview of all the issues and actions related to
its assets:
Locations form the core of the asset monitoring platform. At each location, measurements are recorded. In our
example, a monitoring environment with the name Infrastructure company ACME will be created (Figure 1). We
will create a location ACME Headquarters where we will connect a device, Dragino - N95S31B temperature &
humidity sensor. Each location can consist of multiple location ports. These are ports onto which measurements
are recorded. Here, we create a location port Entrance where the sensor is located. The device can be assigned
several channels that can be linked with any location port. This way, the right measurements can be pushed to
desired locations
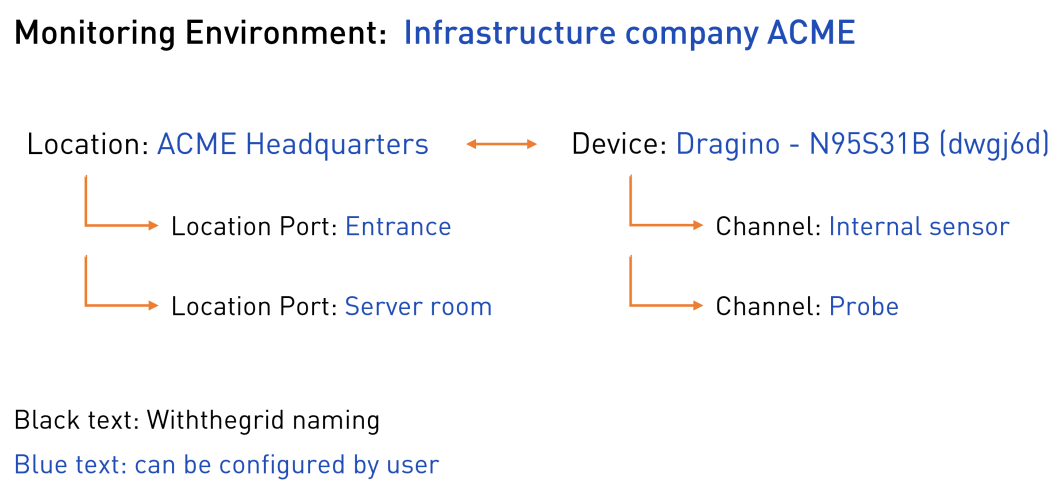
Figure 1. Example of a setup of monitoring environment
- Connectivity environment: Where users configure their devices or data streams and assign them to one or
multiple monitoring environments.
In the connectivity environment, you can configure secure access for your devices through HTTPS webhooks or
client certificates. You can define event handlers in TypeScript that parse incoming payloads into measurement
reports, return instructions to devices and monitor device health.
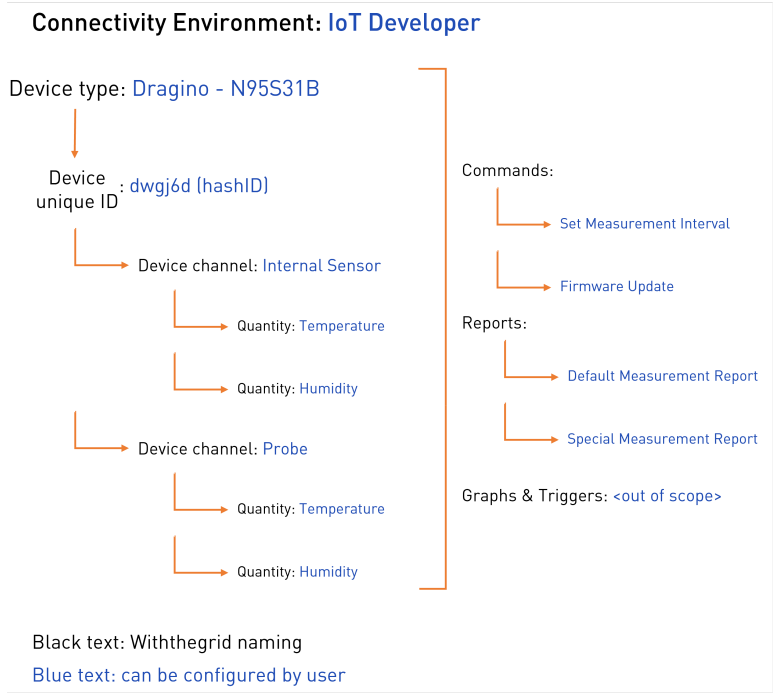
Figure 2. Example of the setup of connectivity environment
In the following example, temperature & humidity sensor Dragino - N95S31B will be connected to the platform.
Every instance of this device type (the physical device) will obtain a unique device ID by the platform which we call
a hashID. Each device can have multiple device channels where measurements are pushed. An example of a
device channel is Internal Sensor. A user can define which measurements will be stored in this channel. Since we
are working with a temperature & humidity, these will be the quantities stored in the channel. Custom commands
can be defined in the connectivity environments such as a request to change the measurement interval of a
connected device but this is out of the scope of this tutorial. Finally, the connectivity environment is used to define
the structure of measurement reports.
Simplified dataflow model
A simplified dataflow model is shown in Figure 3. An HTTP request from IoT Creators is processed by the
webhook. Webhooks identify the communicating device type of the device, it’s unique identifier (e.g. IMEI
number) and forward this information together with the payload to the corresponding device type event handler.
Subsequently, the event handler runs a report parser. The platform offers full flexibility on how to parse payload
into condition reports, using a custom Typescript parser function. The parser should then convert the payload
string (probably applying JSON.parse first) into an actual report with measurements.
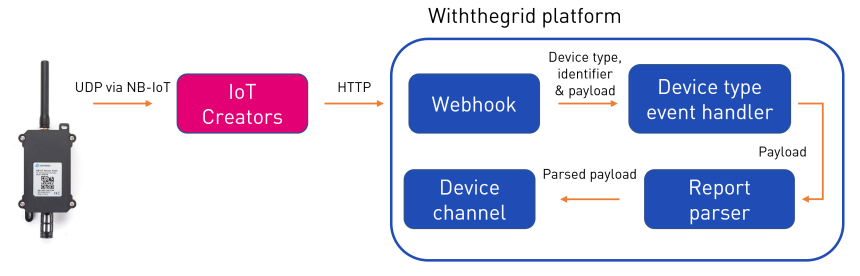
Figure 3. Simplified dataflow model of the Withthegrid platform
Step 1: Creating environments (monitoring + connectivity)
To create a new connectivity environment, simply enter the desired name and select CREATE.
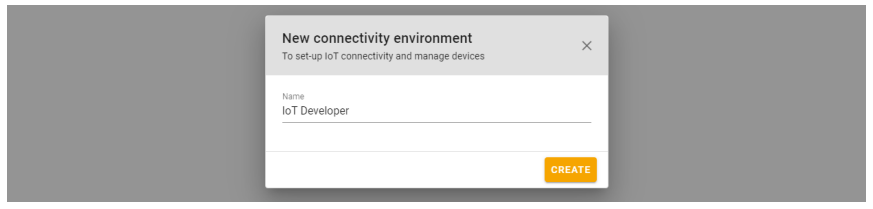
The same step can be followed to create a new monitoring environment. To create the first location in the
monitoring environment, locate the desired place on the map and Add new location.
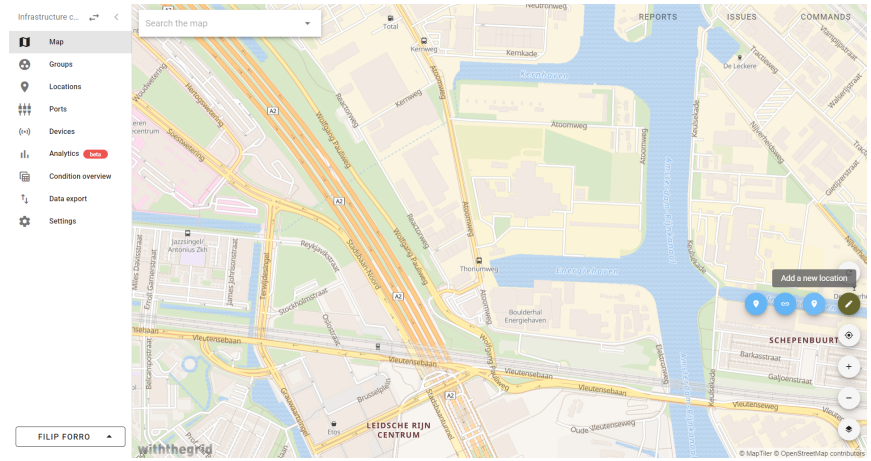
To navigate between the environments, click on the arrows located in the top left corner.
Step 2: Creating and configuring a new webhook
The first step in integrating the Withthegrid platform with IoT creators is to create a new webhook. Webhooks can
be found in the connectivity environment, under Device access/Webhooks. To create a new webhook, click + in
the right bottom corner.
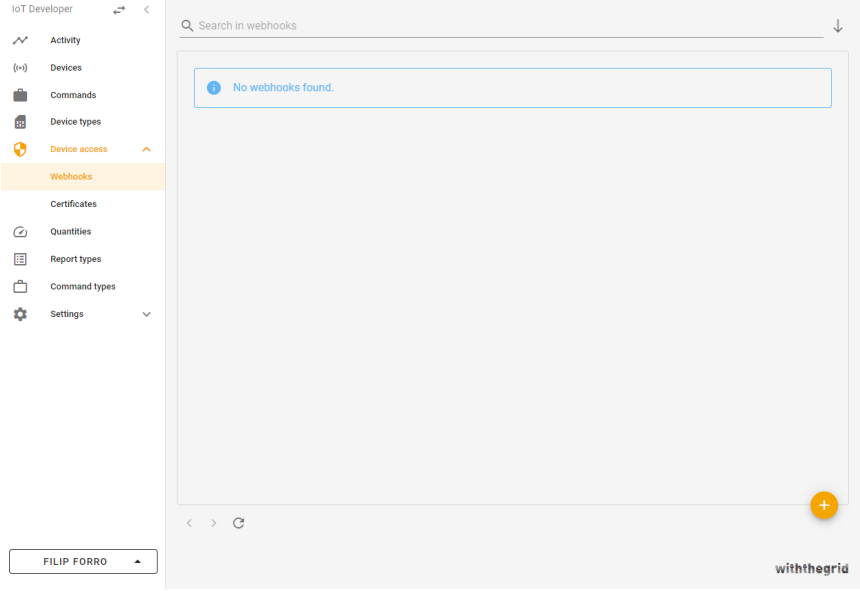
Select the desired webhook name and click ADD.
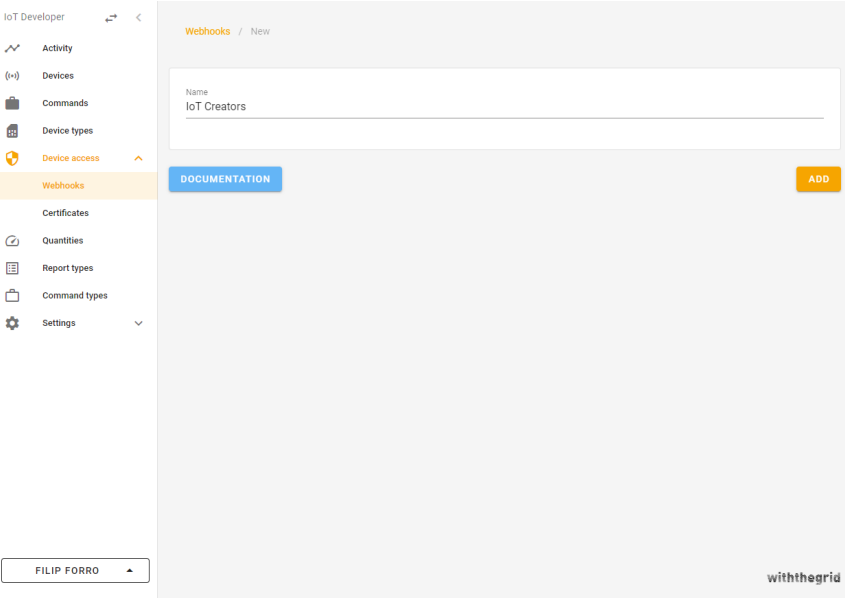
The platform will generate a webhook URL which we can use later to connect with IoT Creators.
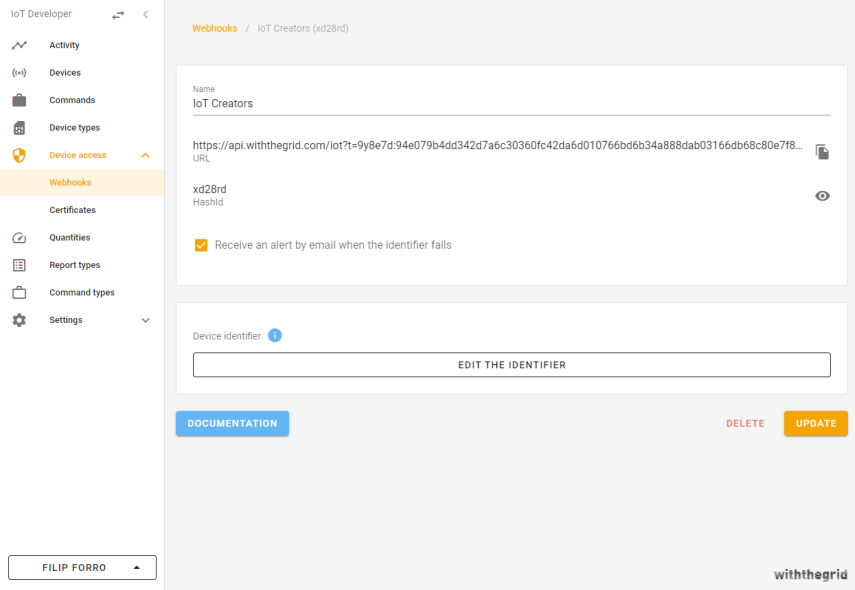
Step 3: Creating and configuring a new device type
A new device type can be created in a connectivity environment by navigating to Device types. This page shows
an overview of all the device types that are available in your environment. Let’s create the first device type by
clicking + in the bottom right corner.
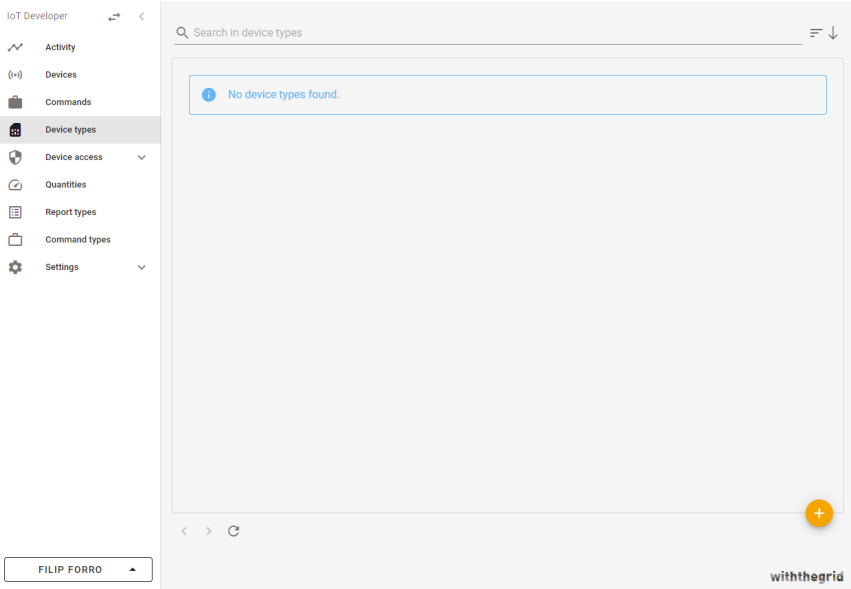
Name the new device type and click ADD. At this point, we can also change the default name of the device
channel to Internal Sensor. This is where humidity and temperature measurements will be pushed.
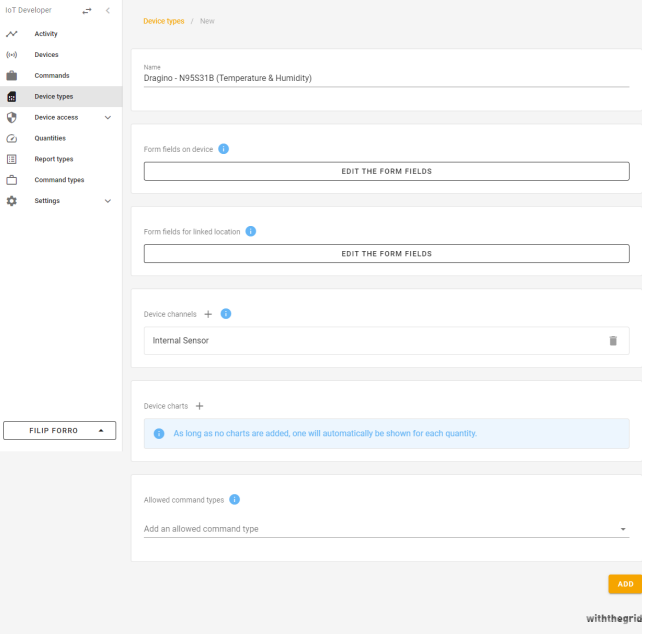
Our new event handler will validate the incoming request and pass the payload to the corresponding report parser
which will parse incoming payloads into measurement reports. The new device type will be given a new hashID
(e.g. 6dkn6y) which is a unique ID that identifies the event handler of Dragino - N95S31B.

Step 4: Creating a new report type and quantities
For each report, a report parser can parse the incoming payload. To create a new report, click + in the bottom right
corner.
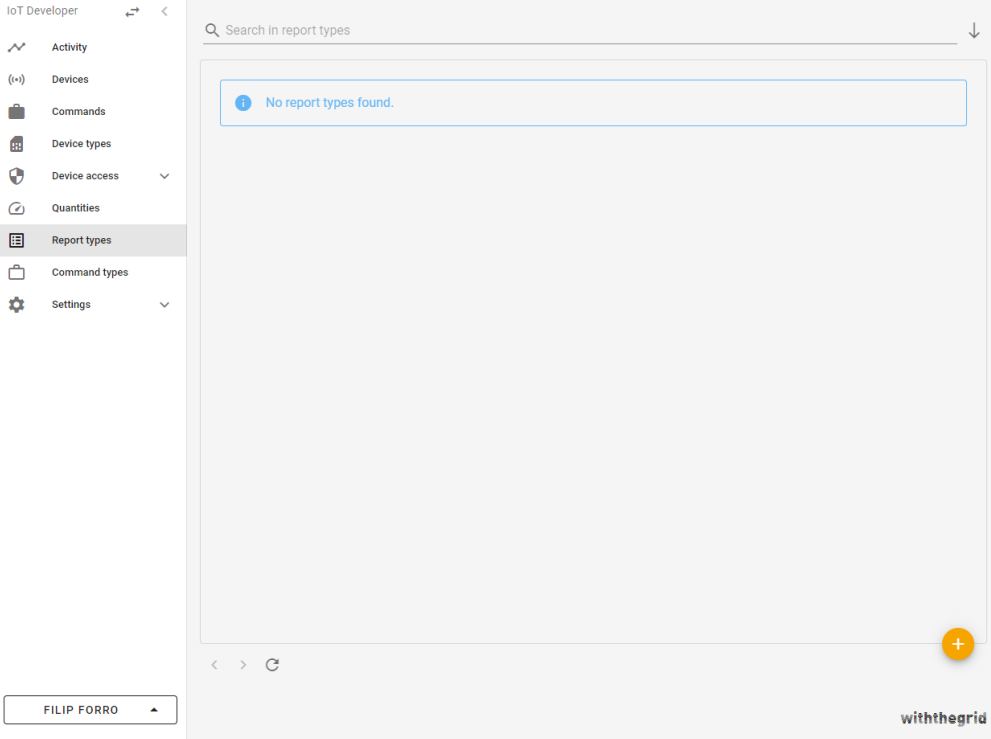
Follow the same steps as before to ADD the new report type.
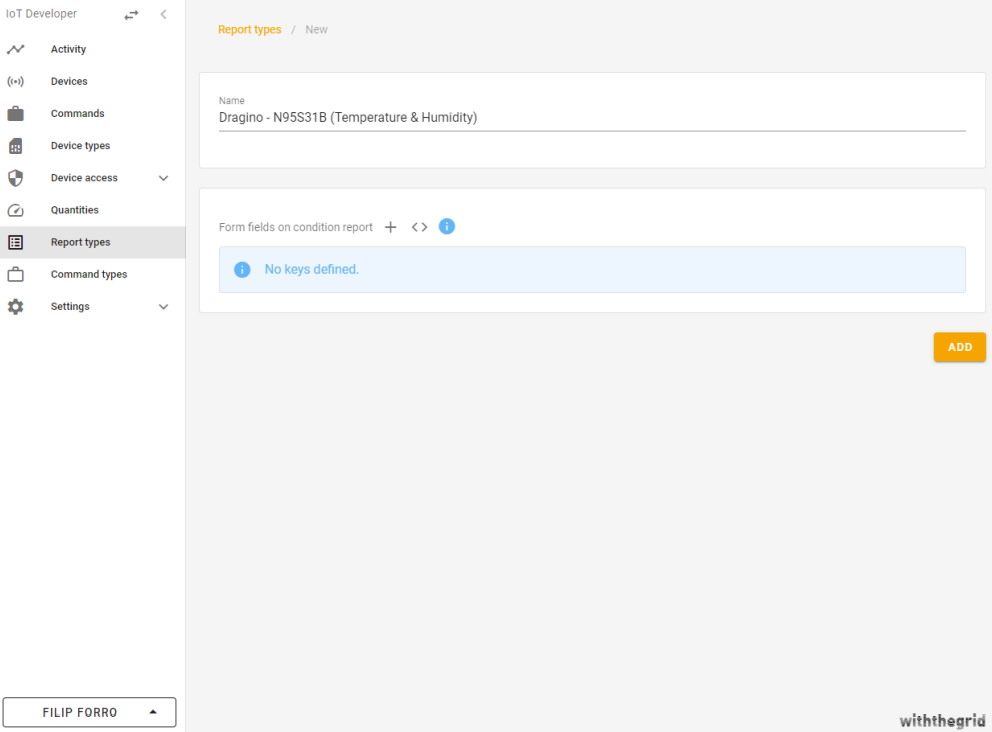
In the report parser, we will correctly parse the incoming payload into a measurement report. A new report type
will be given a hashID (e.g. wyvm7d). Note that the hashID is automatically created and will be different for
everyone.
The quantities of interest in this example are temperature and humidity. Custom quantities can be found in the
panel under option Quantities. The quantities of interest that need to be created are temperature and humidity. To
create a new quantity, select +.
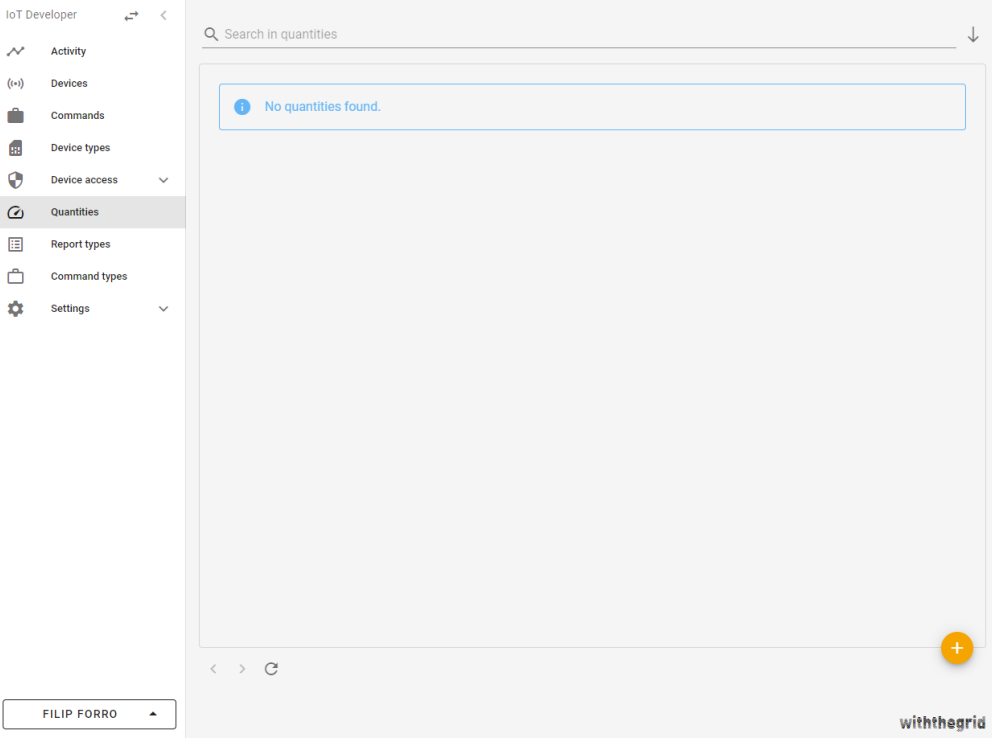
Enter the quantity name (Temperature) and unit (°C). To create the quantity, select ADD.
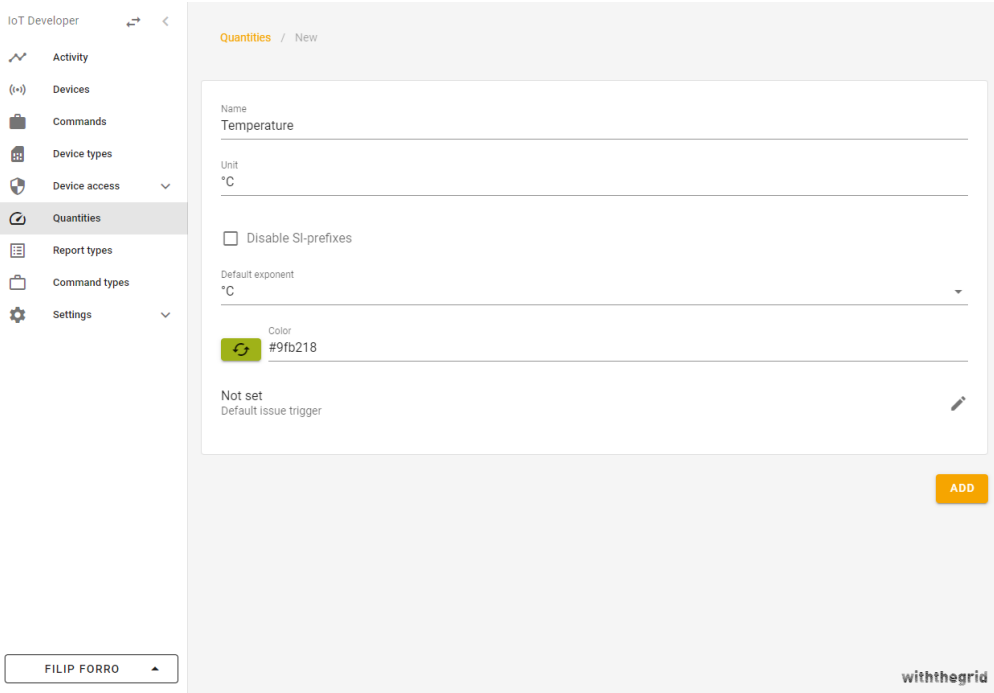
After selecting ADD, a hashID will be created for the newly created quantity. We will create a Humidity quantity by
following the same procedure. In this example, the humidity and temperature hashIDs are yve67d and d2v3qd
respectively. The hashIDs will be used in the report to identify the correct measurement quantity.
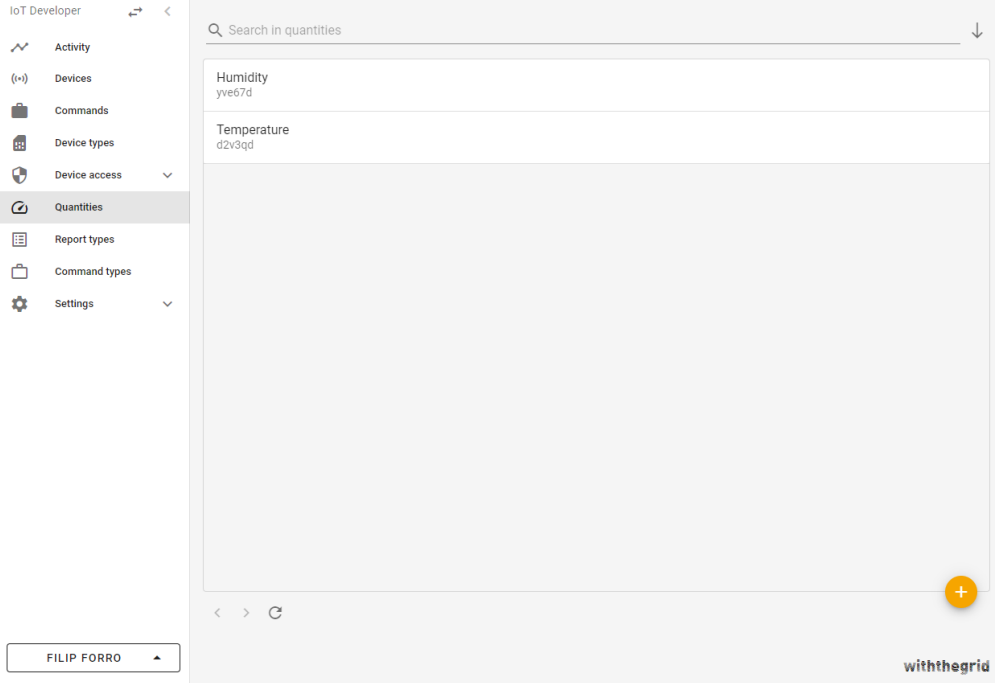
Step 5: Configuring new webhook
The webhook can be edited by navigating to webhooks, selecting the newly created IoT Creators webhook, and
clicking EDIT THE IDENTIFIER. The platform provides a template and a direct link to the webhook
documentation. The following code will take care of IoT Creators registration the first time the webhook URL is
registered at the portal. Afterward, the webhook will pass the payload, together with the device type hashID (which
we will create in the next step) to the appropriate event handler. Lastly, the webhook will also return a
deviceIdentifier as the IMEI of the device.
/**
* This identifier assumes that the device presents its identifier in
* payload.reports[0].serialNumber and that the deviceType is 6dkn6y
* (Dragino - N95S31B)
*/
function handle(args: Arguments): Result | undefined {
// Dragino - N95S31B device type hashID
const deviceType = '6dkn6y';
if(args.request.body == undefined) {
throw new Error('Request body absent');
}
const payload = JSON.parse(JSON.stringify(args.request.body?.data))
if(payload.reports.length === 0) {
// IoT Creators Webhook is registering itself
return {
deviceTypeHashId: deviceType,
deviceIdentifier: 'iot-creators-registration',
};
}
// set the deviceIdentifier to the IMEI of a device
let deviceSerial = payload.reports[0].serialNumber;
deviceSerial = deviceSerial.replace(/^IMEI:/, '');
return {
deviceTypeHashId: deviceType,
deviceIdentifier: deviceSerial,
};
}
Step 6: Configuring device type
The device type that we have created will forward the payload to the report type (which we will create in step 8)
where it will be parsed. The following code will respond to the registration request posted by the IoT Creators
platform. Afterward, it will forward the actual payload generated by Dragino - N95S31B to the report parser.
// define the hashId of the reportType
// will be known at step 8
const reportTypeHashId = 'wyvm7d';
/**
* Handle is the required function. It has two overloads, one for incoming HTTP requests and one for internal events
* When this function is called for an incoming HTTP requests, the function should return information about the response
* that should be returned.
*/
function handle(args: ArgumentsForIncomingRequestEvent, exec: Exec): IncomingRequestResponse;
function handle(args: ArgumentsForEventWithoutResponse, exec: Exec): void;
function handle(args: Arguments, exec: Exec): IncomingRequestResponse | void {
if (args.event.type === 'incomingRequest') {
const request = args.event.webRequest;
if (request.method === 'POST' && request.url.path === '/') {
if (args.device.supplierDeviceIdentifier === 'iot-creators-registration') {
return { statusCode: 204 };
}
// the device is submitting a condition report
if (request.body === undefined || request.body.type !== 'json') {
return {
statusCode: 400,
body: ['parameter_error', 'Body is not of type JSON'],
};
}
// Set receive timestamp
let generatedAt = new Date();
generatedAt.setMilliseconds(0);
// pass parsing of the report to the right report type parser
exec.parseReport({
reportTypeHashId,
payload: JSON.stringify({
generatedAt,
payload: request.body.data,
}),
});
return { statusCode: 204 };
}
return {
statusCode: 404,
body: ['not_found_error', 'Route cannot be found'],
};
}
}
Step 7: Configuring IoT Creators and registering the device
Now that the webhook and the event handler of Dragino - N95S31B are configured, we can set up IoT Creators
project. The first step is to register a device with IMEI on IoT Creators. After the device is registered, we can
configure the application server by copying the webhook URL we created earlier into the callback URL on IoT
Creators.
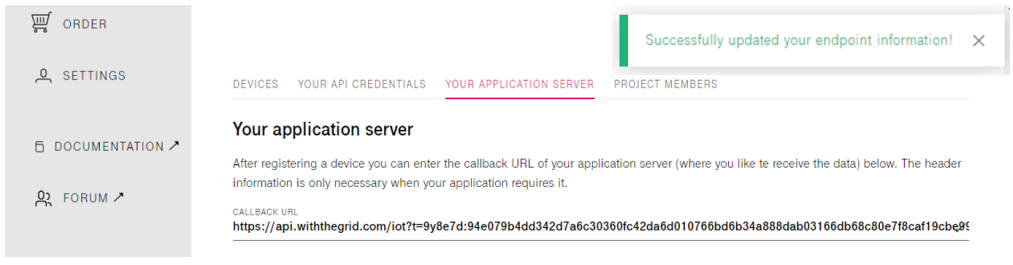
A pop-up on IoT Creators will indicate that the link was successful. This can also be seen in Activity on the
Withthegrid platform while any failed attempts will be marked red.
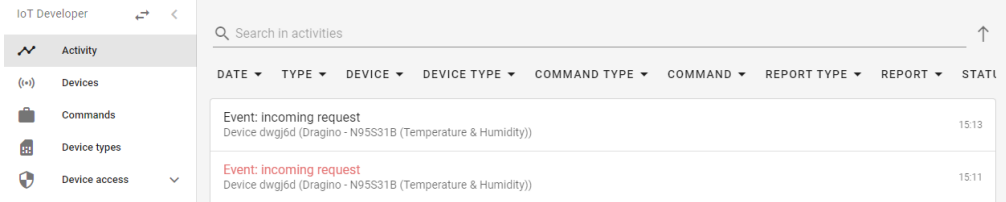
Step 8: Configuring report type
The next step is to correctly configure the created report type such that it can decode the incoming payload into
physical measurements (temperature & humidity). The following report parser decodes the Dragino - N95S31B
humidity and temperature measurement and pushes them to the Internal Sensor channel which is the first
channel, therefore, has a channelIndex of 0.
// define the has ID of quantities of interest
const quantityHashIds = {
temperature: 'd2v3qd',
humidity: 'yve67d'
};
function handle(args: Arguments, exec: Exec): Result {
const data = JSON.parse(args.payload);
if (typeof data !== 'object' || data === null) {
throw new Error('Data is not a JSON-object');
}
const generatedAtMs = Date.parse(data.generatedAt);
if (Number.isNaN(generatedAtMs)) {
throw new Error('generatedAt cannot be parsed into a valid Date');
}
const generatedAt = new Date(generatedAtMs);
const hexstr = data.payload.reports[0].value;
const payload = stringToBytes(hexstr);
let temp_raw = ((payload[17] << 8) + payload[18]);
const temp = ( (temp_raw & 0xf000) ? ((temp_raw & 0x0FFF) * -1) : temp_raw);
const hum = ((payload[19] << 8) + payload[20]);
const measurements: Result['measurements'] = [];
// Measurements are registered using scientific notation, so you provide integer
// significand and an orderOfMagnitude. The value can then be calculated as
// significand * 10 ** orderOfMagnitude.
if (temp !== null) {
measurements.push({
channelIndex: 0, // Internal Sensor channel
quantityHashId: quantityHashIds.temperature, // measurement quantity hash ID
generatedAt, // timestemp
significand: temp,
orderOfMagnitude: -1, // 225 = 22.5 deg C
});
}
if (hum !== null) {
measurements.push({
channelIndex: 0,
quantityHashId: quantityHashIds.humidity,
generatedAt,
significand: hum,
orderOfMagnitude: -1, // 390 = 39.0 %rh
});
}
return {
generatedAt,
measurements,
fields: {}
};
}
function stringToBytes(hex: string) {
for (var bytes = [], c = 0; c < hex.length; c += 2) {
bytes.push(parseInt(hex.substr(c, 2), 16));
}
return bytes;
}
Step 9: Claiming device tokens
When the N95S31B sensor is linked for the first time, the instance of the device is created in the platform. This
can be found in Devices. The corresponding device will have a unique hashID (e.g. dwgj6d), Identifier (in our
case IMEI of the device), and will be of device type: Dragino - N95S31B. The device can be easily placed in any
monitoring environment by selecting GET CLAIM TOKEN(S) in the bottom right corner.
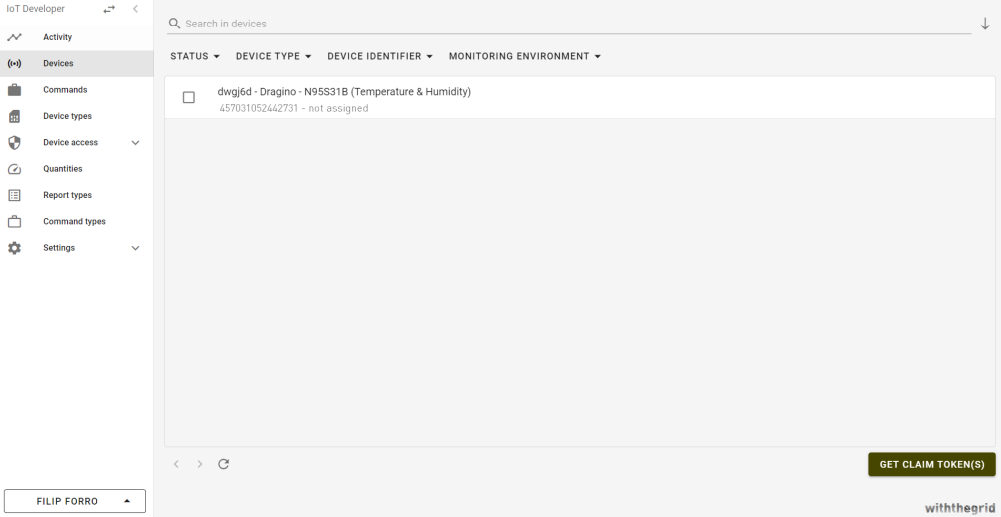
Enter the hashID of a device to be claimed and click REQUEST.
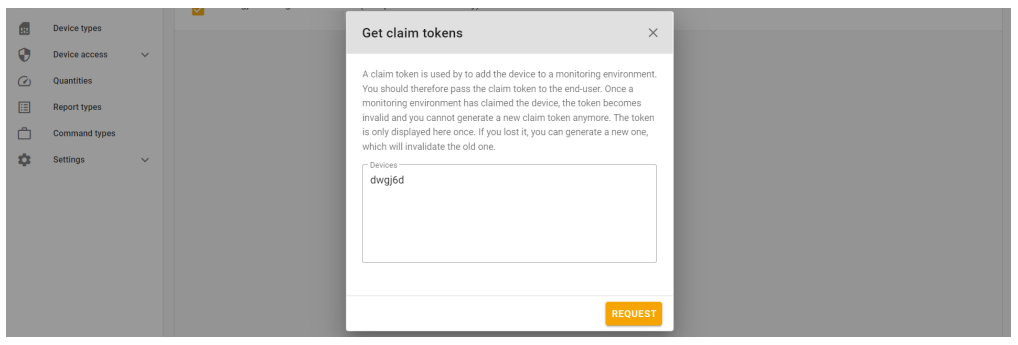
The platform will output a claim token that can be copied.
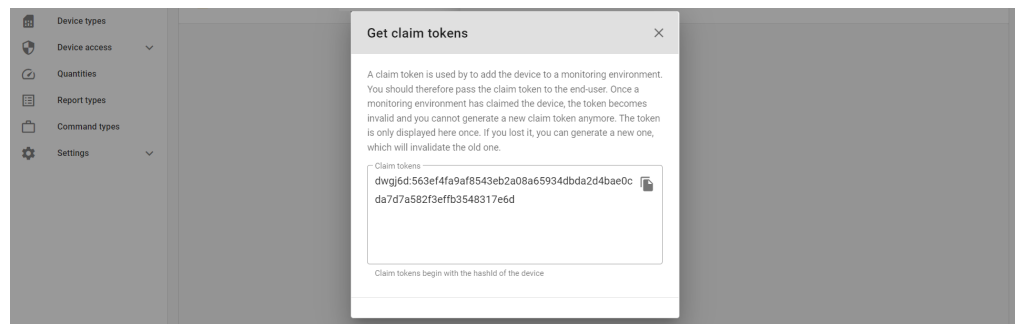
In the last step, we will configure the monitoring environment and add the newly connected sensor.
Step 10: Configuring the monitoring environment
To add a device into the monitoring environment, navigate to Devices, click +, and enter the previously copied
claim token. Click ADD.
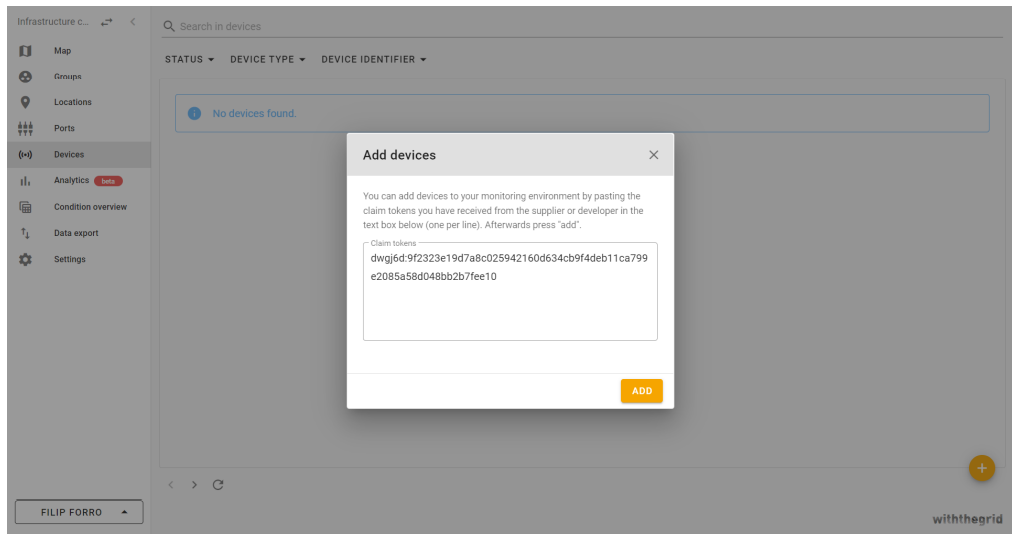
The following image shows that the device is registered in the monitoring environment, however, is not installed
yet.
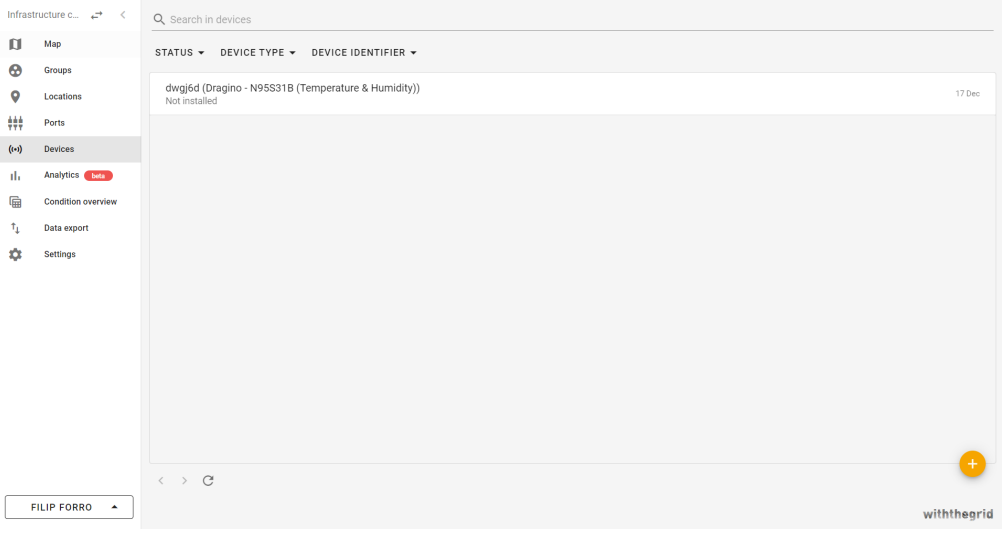
To install a device, navigate to the created location (ACME Headquarters) and select Devices.
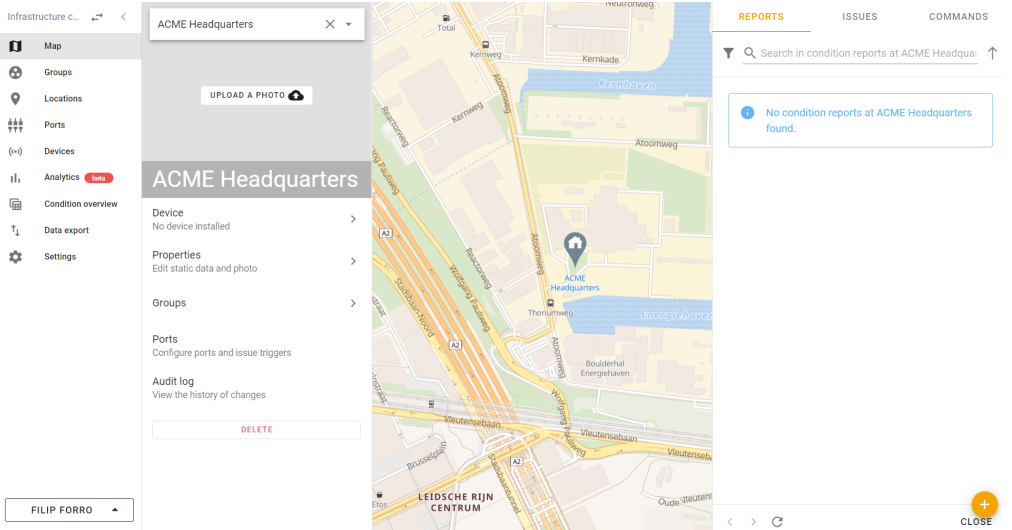
In Link to a device, select the Dragino - N95S31B. Finally, we would like to track the Internal Sensor port onto
which measurements are pushed. Click +PORT to select the port with temperature and humidity measurements.
Finalize the device installation with SET.
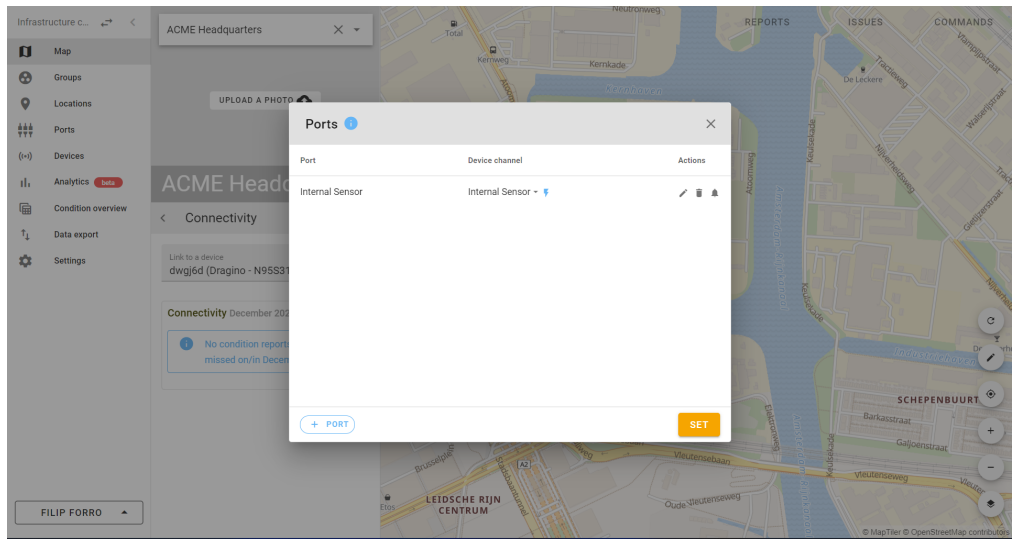
From this point on, all measurements pushed to the Internal Sensor channel of Dragino - N95S31B sensor with
IMEI: 457031052442731 will be available in the monitoring environments ready to be visualized in graphs.
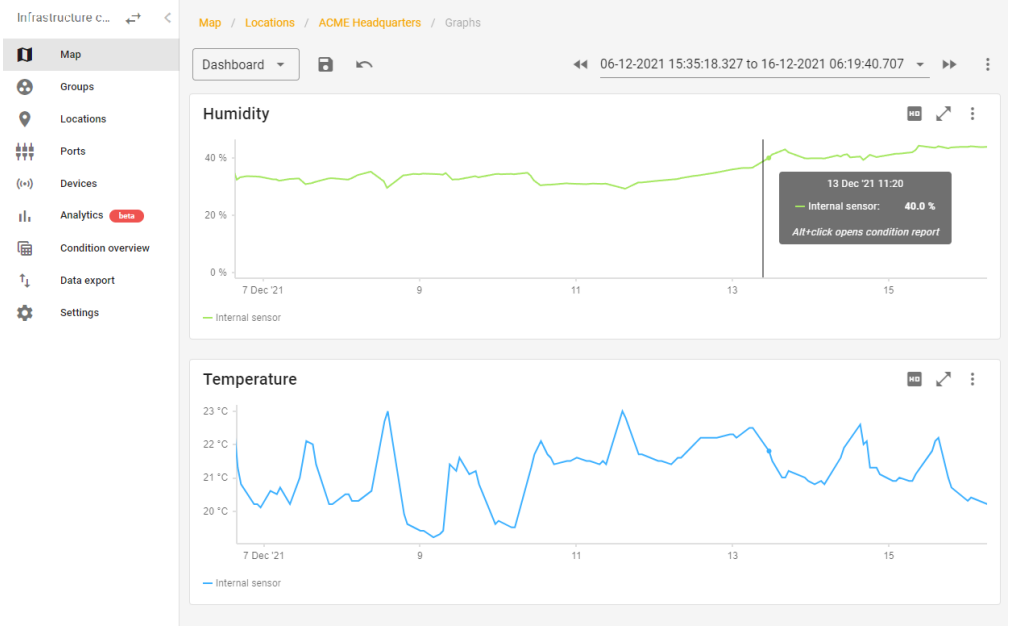
Congratulations! If you can see measurements in the graphs, you have successfully finished the tutorial. If you
want to learn more about all the cool functionalities that the Withthegrid platform offers, do not hesitate to look at
their documentation or contact them directly through withthegrid.com
Updated over 2 years ago